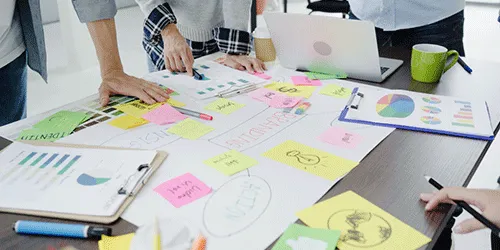
Plataforma de Marketing
Realiza campañas por SMS Masivo, Email Marketing, Voz y mucho más
Envíe SMS o SMS Masivo a través de web, PC o API
Conecte con sus clientes con WhatsAPP Business
Envíe llamadas de voz de forma automatizada
Comunicaciones Certificadas con validez legal
Ofrezca soporte a través de Chat Web
Realiza campañas por SMS Masivo, Email Marketing, Voz y mucho más
Mejore la seguridad de sus sistemas para sus clientes
Automatice las campañas de marketing de su empresa
Automatice el soporte técnico de su empresa
Envíe y firme contratos de forma digital
Envíe encuestas de satisfacción a sus clientes
Cree y envíe magnificas landing pages a sus clientes
Gestione y envíe cupones de descuento a sus clientes